I’ve bought myself an Alienware m5500, which is a laptop based on the Uniwill 259EN3. I wanted a laptop with a high resolution screen (the m5500 does 1920×1200 on a 15″ LCD) and Intel graphics. (I don’t want to support either ATI or nVidia, and IBM and Dell make you choose an ATI/nVidia card if you go above their standard resolution screens). My machine has:
- Intel Pentium M 730 1.6GHz 2MB L2 Cache 533MHz FSB
- 512M RAM, 40G disk
- Alienware m5500 15.4″ WideUXGA 1920×1200 LCD
- Intel GMA900 (915GM/i810) Extreme Graphics
- Intel PRO/Wireless 2200 b/g Wireless Card
I have Fedora Core 5 running on it now, but I’m sad to say that it wasn’t without pain:
I booted from the FC5 install CD. The kernel booted, switched over to Anaconda, and I got a black screen and hung system. Tried again, this time using these instructions to start a VNC installer. That worked, and I soon found out that Fedora now has no NTFS support at all, which meant that the single NTFS partition taking up the entire disk would have to be deleted. (Which sounded fine at the time but is annoying me now — see the wifi section below.) Ubuntu’s installer can do NTFS resizing, but Fedora thinks that the kernel NTFS support violates patents and is not legally redistributable.
After the install, I got the same X crash on my first boot. Booting into runlevel 3 got me to a shell. I determined that the X failure was this bug, which requires applying this patch to Xorg to get the card working. Now that Xorg is modular, it’s (thankfully) sufficient to just download the latest xorg-x11-server.src.rpm, make the change, and rpmbuild -ba
to have the specfile take care of building you a new RPM.
That got X working at 800×600; to get to 1920×1200 you need to use 915resolution and the following modeline:
ModeLine "1920x1200" 230 1920 1936 2096 2528 1200 1201 1204 1250
Now, on to wireless. I downloaded the ieee80211 stack, ipw2200 driver and ipw2000 firmware, and:
unity:cjb~ % sudo modprobe ipw2200
ipw2200: Detected Intel PRO/Wireless 2200BG Network Connection
ipw2200: Radio Frequency Kill Switch is On:
Kill switch must be turned off for wireless networking to work.
ipw2200: Detected geography ZZM (11 802.11bg channels, 0 802.11a channels)
There is a wifi button on the case, next to the power switch — which implies that it’s a hardware button, rather than a software button like the function keys on the keyboard — but pressing it turns on the “sleep” LED rather than the “wifi” LED, and ipw2200 continues to make the same complaint. I see that drivers exist to control the kill switch in software, but none I’ve seen work on this machine. I suspect that booting into Windows (which I can’t do now since I wasn’t able to keep/resize the partition) would get the wifi working in Linux either temporarily or permanently. I took a backup of the disk (dd if=/dev/sda of=nfs-mounted-backup
) and booted the XP recovery CD that came with the machine, but it either hung or needed many minutes at “Setup is inspecting your computer’s hardware configuration”, and my patience for Windows is pretty limited. I’ll try again if I don’t get any other suggestions.
I posted some cries for help about this and dug out my very old, trusty Orinoco wireless PC Card and inserted it. And the machine hung immediately, and did so repeatably. I dug around until I found this linux-kernel thread which contains a safer /etc/pcmcia/config.opts
, and that’s working fine. Update: Hmph. It works anytime after init has loaded (udev, perhaps?), but the machine hangs at the cs: memory probe
if I try to boot with the card already inserted. Any ideas?
I set about installing AIGLX, and the performance is good, especially for an Intel chipset. The photo at the bottom shows AIGLX and true transparency in gnome-terminal, which is lovely to have (at last), especially on a laptop when you’re likely to want to be copying from a web page into a terminal, etc.
So, that’s about it for day one. To summarise:
ACPI
Well, sleep (suspend-to-ram; S3) seemed to be working out of the box, but it isn’t now. It looks like the IDE driver isn’t coming back; if I can keep an ssh session connected, I see repeated in dmesg:
sd 0:0:0:0: SCSI error: return code = 0x40000
end_request: I/O error, dev sda, sector 18800717
I’ll look into having the sd module removed and restarted — anyone know how I should do that on FC5/Rawhide? I also had to change resume_video() in /etc/pm/functions-intel to get video back:
#/usr/sbin/vbetool post
#/usr/sbin/vbetool vbestate restore < /var/run/vbestate
/usr/sbin/vbetool dpms on
Suspend to disk works, and doesn't even take that long, but kicks me back to the gdm prompt (!) from a logged in session. Update: Working now, after putting 915resolution in the resume path as well. Thanks, Jens!
The ACPI function keys don't do anything in software, not sure which driver they need.
CPU scaling
Working, switches between 750MHz/1.1GHz/1.6GHz automatically.
Graphics
Working after patching Xorg, acceleration works, AIGLX works.
Synaptics touchpad
Working, I disabled tap-to-click and vertical scrolling:
Option "VertScrollDelta" "0"
Option "HorizScrollDelta" "0"
Option "MaxTapMove" "0"
Option "MaxTapTime" "0"
USB, Sound (snd-intel-hda), SD card reader, DVD burning, Firewire.
Working.
VGA out
Works, after adding the following:
Option "MonitorLayout" "CRT,LFP"
Option "Clone" "true"
Option "SWCursor" "true"
I'll keep this post updated as I learn more about the machine. Here's the photo:
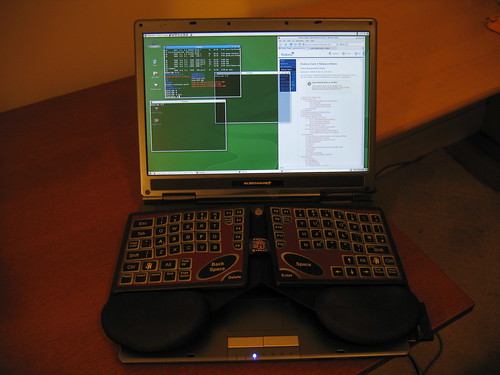